Install node and npm from After installation in VSCode under terminal tab run node Paste your snippet to run your javascript code or use node filepath/filename.js to see result. Using React in Visual Studio Code. React is a popular JavaScript library developed by Facebook for building web application user interfaces. The Visual Studio Code editor supports React.js IntelliSense and code navigation out of the box. Welcome to React.
React is a popular JavaScript library developed by Facebook for building web application user interfaces. The Visual Studio Code editor supports React.js IntelliSense and code navigation out of the box.
Welcome to React
We'll be using the create-react-app
generator for this tutorial. To use the generator as well as run the React application server, you'll need Node.js JavaScript runtime and npm (Node.js package manager) installed. npm is included with Node.js which you can download and install from Node.js downloads.
Tip: To test that you have Node.js and npm correctly installed on your machine, you can type node --version
and npm --version
in a terminal or command prompt.
You can now create a new React application by typing:
where my-app
is the name of the folder for your application. This may take a few minutes to create the React application and install its dependencies.
Note: If you've previously installed create-react-app
globally via npm install -g create-react-app
, we recommend you uninstall the package using npm uninstall -g create-react-app
to ensure that npx always uses the latest version.
Let's quickly run our React application by navigating to the new folder and typing npm start
to start the web server and open the application in a browser:
You should see the React logo and a link to 'Learn React' on http://localhost:3000 in your browser. We'll leave the web server running while we look at the application with VS Code.
To open your React application in VS Code, open another terminal or command prompt window, navigate to the my-app
folder and type code .
:
Markdown preview
In the File Explorer, one file you'll see is the application README.md
Markdown file. This has lots of great information about the application and React in general. A nice way to review the README is by using the VS Code Markdown Preview. You can open the preview in either the current editor group (Markdown: Open Preview⇧⌘V (Windows, Linux Ctrl+Shift+V)) or in a new editor group to the side (Markdown: Open Preview to the Side⌘K V (Windows, Linux Ctrl+K V)). You'll get nice formatting, hyperlink navigation to headers, and syntax highlighting in code blocks.
Syntax highlighting and bracket matching
Now expand the src
folder and select the index.js
file. You'll notice that VS Code has syntax highlighting for the various source code elements and, if you put the cursor on a parenthesis, the matching bracket is also selected.
IntelliSense
As you start typing in index.js
, you'll see smart suggestions or completions.
After you select a suggestion and type .
, you see the types and methods on the object through IntelliSense.
VS Code uses the TypeScript language service for its JavaScript code intelligence and it has a feature called Automatic Type Acquisition (ATA). ATA pulls down the npm Type Declaration files (*.d.ts
) for the npm modules referenced in the package.json
.
If you select a method, you'll also get parameter help:
Go to Definition, Peek definition
Through the TypeScript language service, VS Code can also provide type definition information in the editor through Go to Definition (F12) or Peek Definition (⌥F12 (Windows Alt+F12, Linux Ctrl+Shift+F10)). Put the cursor over the App
, right click and select Peek Definition. A Peek window will open showing the App
definition from App.js
.
Press Escape to close the Peek window.
Hello World!
Let's update the sample application to 'Hello World!'. Create a new H1 header with 'Hello, world!' and replace the <App />
tag in ReactDOM.render
with element
.
Once you save the index.js
file, the running instance of the server will update the web page and you'll see 'Hello World!' when you refresh your browser.
Tip: VS Code supports Auto Save, which by default saves your files after a delay. Check the Auto Save option in the File menu to turn on Auto Save or directly configure the files.autoSave
user setting.
Debugging React
To debug the client side React code, we'll need to install the Debugger for Chrome extension.
Note: This tutorial assumes you have the Chrome browser installed. There are also debugger extensions for the Edge and Firefox browsers.
Open the Extensions view (⇧⌘X (Windows, Linux Ctrl+Shift+X)) and type 'chrome' in the search box. You'll see several extensions which reference Chrome.
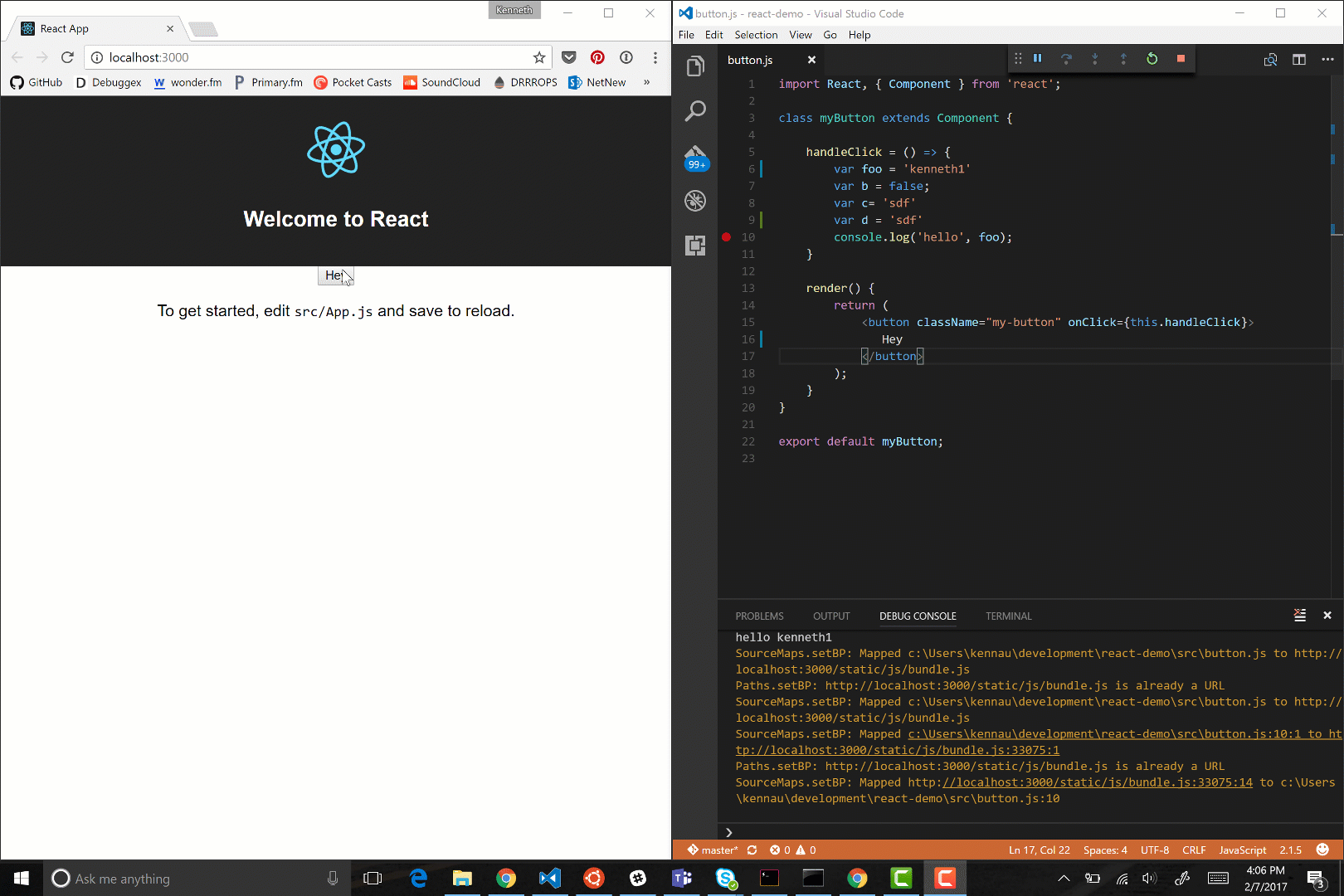
Press the Install button for Debugger for Chrome.
Set a breakpoint
To set a breakpoint in index.js
, click on the gutter to the left of the line numbers. This will set a breakpoint which will be visible as a red circle.
Configure the Chrome debugger
We need to initially configure the debugger. To do so, go to the Run view (⇧⌘D (Windows, Linux Ctrl+Shift+D)) and click create a launch.json file to customize Run and Debug. Choose Chrome from the Select Environment dropdown list. This will create a launch.json
file in a new .vscode
folder in your project which includes a configuration to launch the website.
We need to make one change for our example: change the port of the url
from 8080
to 3000
. Your launch.json
should look like this:
Ensure that your development server is running (npm start
). Then press F5 or the green arrow to launch the debugger and open a new browser instance. The source code where the breakpoint is set runs on startup before the debugger was attached, so we won't hit the breakpoint until we refresh the web page. Refresh the page and you should hit your breakpoint.
You can step through your source code (F10), inspect variables such as element
, and see the call stack of the client side React application.
The Debugger for Chrome extension README has lots of information on other configurations, working with sourcemaps, and troubleshooting. You can review it directly within VS Code from the Extensions view by clicking on the extension item and opening the Details view.
Live editing and debugging
If you are using webpack together with your React app, you can have a more efficient workflow by taking advantage of webpack's HMR mechanism which enables you to have live editing and debugging directly from VS Code. You can learn more in this Live edit and debug your React apps directly from VS Code blog post and the webpack Hot Module Replacement documentation.
Linting
Visual Studio Code Run Javascript File
Linters analyze your source code and can warn you about potential problems before you run your application. The JavaScript language services included with VS Code has syntax error checking support by default, which you can see in action in the Problems panel (View > Problems⇧⌘M (Windows, Linux Ctrl+Shift+M)).
Try making a small error in your React source code and you'll see a red squiggle and an error in the Problems panel.
Linters can provide more sophisticated analysis, enforcing coding conventions and detecting anti-patterns. A popular JavaScript linter is ESLint. ESLint, when combined with the ESLint VS Code extension, provides a great in-product linting experience.
First, install the ESLint command-line tool:
Then install the ESLint extension by going to the Extensions view and typing 'eslint'.
Once the ESLint extension is installed and VS Code reloaded, you'll want to create an ESLint configuration file, .eslintrc.js
. You can create one using the extension's ESLint: Create ESLint configuration command from the Command Palette (⇧⌘P (Windows, Linux Ctrl+Shift+P)).
The command will prompt you to answer a series of questions in the Terminal panel. Take the defaults, and it will create a .eslintrc.js
file in your project root that looks something like this:
ESLint will now analyze open files and shows a warning in index.js
about 'App' being defined but never used.
You can modify the ESLint rules in the .eslintrc.js
file.
Let's add an error rule for extra semi-colons:

Now when you mistakenly have multiple semicolons on a line, you'll see an error (red squiggle) in the editor and error entry in the Problems panel.
Popular Starter Kits
In this tutorial, we used the create-react-app
generator to create a simple React application. There are lots of great samples and starter kits available to help build your first React application.
VS Code React Sample
This is a sample React application used for a demo at the 2016 //Build conference. The sample creates a simple TODO application and includes the source code for a Node.js Express server. It also shows how to use the Babel ES6 transpiler and then use webpack to bundle the site assets.
TypeScript React
If you're curious about TypeScript and React, you can also create a TypeScript version of the create-react-app
application by specifying that you want to use the TypeScript template:
See the details at Adding TypeScript on the Create React App site.
Angular
Angular is another popular web framework. If you'd like to see an example of Angular working with VS Code, check out the Chrome Debugging with Angular CLI recipe. It will walk you through creating an Angular application and configuring the launch.json
file for the Debugger for Chrome extension.
Common questions
Can I get IntelliSense within declarative JSX?
Yes. For example, if you open the create-react-app
project's App.js
file, you can see IntelliSense within the React JSX in the render()
method.
How To Run Javascript In Visual Code Studio
-->You can use the JavaScript Console window to interact with and debug UWP apps built using JavaScript. These features are supported for UWP apps and apps created using Visual Studio Tools for Apache Cordova. For the console command reference, see JavaScript Console commands.
The JavaScript Console window allows you to:
Send objects, values, and messages from your app to the console window.
View and modify the values of local and global variables in the running app.
View object visualizers.
Run JavaScript code that executes within the current script context.
View JavaScript errors and exceptions, in addition to Document Object Model (DOM) and Windows Runtime exceptions.
Perform other tasks, like clearing the screen. See JavaScript Console commands for the full list of commands.
Tip
If the JavaScript Console window is closed, choose Debug> Windows > JavaScript Console to re-open it. The window appears only during a script debugging session.
Using the JavaScript Console window, you can interact with your app without stopping and restarting the debugger. For more info, see Refresh an app (JavaScript). For info on other JavaScript debugging features, such as using the DOM Explorer and setting breakpoints, see Quickstart: Debug HTML and CSS and Debug apps in Visual Studio.
Debug by using the JavaScript Console window
The following steps create a FlipView
app and show how to interactively debug a JavaScript coding error.
Note
The sample app here is a UWP app. However, the console features described here also apply to apps created using Visual Studio Tools for Apache Cordova.
To debug JavaScript code in the FlipView app
Create a new solution in Visual Studio by choosing File > New Project.
Choose JavaScript > Windows Universal, and then choose WinJS App.
Type a name for the project, such as
FlipViewApp
, and choose OK to create the app.In the BODY element of index.html, replace the existing HTML code with this code:
Open default.css and add the CSS for the
#fView
selector:Open default.js and replace the code with the following JavaScript code:
If a debugging target isn't already selected, choose Local Machine from the drop-down list next to the Device button on the Debug toolbar:
Press F5 to start the debugger.
The app runs but images are missing. APPHOST errors in the JavaScript Console window indicate that images are missing.
With the
FlipView
app running, typeData.items
in the console window input prompt (next to the '>>' symbol) and press Enter.A visualizer for the
items
object appears in the console window. This indicates that theitems
object instantiated and is available in the current script context. In the console window, you can click through the nodes of an object to view property values (or use the arrow keys). If you click down into theitems._data
object, as you see in this illustration, you'll find that its image source references are incorrect, as expected. The default images (logo.png) are still present in the object, and there are missing images interspersed with the expected images.Also note that there are many more items in
items._data
object than you would expect.At the prompt, type
Data.items.push
and press Enter. The console window shows a visualizer for thepush
function, which is implemented in a Windows Library for JavaScript (WinJS) project file. In this app, we are usingpush
to add the correct items. With a little investigation using IntelliSense, we find out that we should be usingsetAt
to replace the default images.To fix this problem interactively without stopping the debugging session, open default.js and select this code from the
updateImages
function:Copy and paste this code into the JavaScript Console input prompt.
Tip
When you paste multiple lines of code into the JavaScript Console input prompt, the console input prompt automatically switches to multiline mode. You can press Ctrl+Alt+M to turn multiline mode on and off. To run a script in multiline mode, press Ctrl+Enter or choose the arrow symbol in the lower-right corner of the window. For more info, see Single-line mode and multiline mode in the JavaScript Console window.
Correct the
push
function calls in the prompt, replacingpages.push
withData.items.setAt
. The corrected code should look like this:Tip
If you want to use the
pages
object instead ofData.items
, you would need to set a breakpoint in your code to keep thepages
object in scope.Choose the green arrow symbol to run the script.
Press Ctrl+Alt+M to switch the console input prompt to single-line mode, and then choose Clear input (the red 'X') to delete the code from the input prompt.
Type
Data.items.length = 3
at the prompt, and then press Enter. This removes the extraneous elements from the data.Check the app again, and you'll see that the correct images are on the correct
FlipView
pages.In DOM Explorer, you can see the updated DIV element, and you can navigate into the subtree to find the expected IMG elements.
Stop debugging by choosing Debug > Stop Debugging or by pressing Shift+F5, and then fix the source code.
For the complete default.html page containing corrected sample code, see Debug HTML, CSS, and JavaScript sample code.
Interactive debugging and break mode

You can use breakpoints and step into code while you're using JavaScript debugging tools like the JavaScript Console window. When a program that's running in the debugger encounters a breakpoint, the debugger temporarily suspends execution of the program. When execution is suspended, your program switches from run mode to break mode. You can resume execution at any time.
When a program is in break mode, you can use the JavaScript Console window to run scripts and commands that are valid in the current script execution context. In this procedure, you'll use the fixed version of the FlipView
app that you created earlier to demonstrate the use of break mode.
To set a breakpoint and debug the app
In the default.html file of the
FlipView
app that you previously created, open the shortcut menu for theupdateImages()
function, and then choose Breakpoint > Insert Breakpoint.Choose Local Machine in the drop-down list next to the Start Debugging button on the Debug toolbar.
Choose Debug > Start Debugging, or press F5.
The app enters break mode when execution reaches the
updateImages()
function, and the current line of program execution is highlighted in yellow.You can change the values of variables to immediately affect the program state without ending the current debugging session.
Type
updateImages
at the prompt and press Enter. A visualizer for the function appears in the console window.Select the function in the console window to show the function implementation.
The following illustration shows the console window at this point.
Copy one line of the function from the output window to the input prompt, and change the index value to 3:
Press Enter to run the line of code.
If you want to step through the code line by line, press F11, or press F5 to continue program execution.
Press F5 to continue program execution. The
FlipView
app appears, and now all four pages show one of the non-default images.To switch back to Visual Studio, press F12 or Alt+Tab.
Single-line mode and multiline mode in the JavaScript Console window
The input prompt for the JavaScript Console window supports both single-line mode and multiline mode. The interactive debugging procedure in this topic provides an example of using both modes. You can press Ctrl+Alt+M to switch between modes.
Single-line mode provides input history. You can navigate through the input history by using the Up Arrow and Down Arrow keys. Single-line mode clears the input prompt when you run scripts. To run a script in single-line mode, press Enter.
Multiline mode does not clear the input prompt when you run scripts. When you switch to single-line mode from multiline mode, you can clear the input line by pressing Clear input (the red 'X'). To run a script in multiline mode, press Ctrl+Enter or choose the arrow symbol in the lower-right corner of the window.
Switching the script execution context
The JavaScript Console window allows you to interact with a single execution context, which represents a single instance of the web platform host (WWAHost.exe), at a time. In some scenarios, your app may start another instance of the host, such as when you use an iframe
, a share contract, a web worker, or a WebView
control. If another instance of the host is running, you can select a different execution context while running the app by selecting the execution context in the Target list.
The following illustration shows the Target list in the JavaScript Console window.
You can also switch the execution context by using the cd
command, but you must know the name of the other execution context and the reference you use must be in scope. The Target list provides better access to other execution contexts.

See also
